The main advantages of containerizing the microservices are
- It makes applications portable, as dependencies can be packaged together with applications. For example, if one application needs JRE7 and the other needs JRE8, they can be packaged separately with their respective dependencies and deployed on a server, regardless of what JRE version exists on the server.
- It removes the bottleneck of being limited by a number of servers during the release process, as you can deploy multiple versions of the same image or multiple features at the same time. For example, you can deploy multiple features for QA at the same time, regardless of how many servers you have.
- You can start multiple instances of the application to handle increasing load.
- As the containers can be isolated from each other, it will help in security.
Docker is a command line program, a background daemon. Docker containers run natively on Linux and share the kernel, making it very light weight as compared to VMs.
In this post, we will containerize a Spring Microservice with Docker. First, install Docker and make sure it is working as listed at Install Docker.
Then go to Spring Initializer site and add Web as a dependency and click on Generate Project to download a template project. Import the project into your favorite IDE and add the @RestController annotation to the main class. Add additional method with @RequestMapping as shown below.
Run the application and go to http://localhost:8080 and you should see “My First Dockerized Microservice” in your browser. This ensures, our service builds and runs fine without Docker. Stop the application and close your IDE.
Add a file called Dockerfile to the project directory and copy and paste the following contents into it.
1 2 3 4 5 |
FROM openjdk:8-jdk-alpine VOLUME /tmp ADD build/libs/microservice-docker-0.0.1-SNAPSHOT.jar app.jar EXPOSE 8080 ENTRYPOINT ["java","-jar","/app.jar"] |
Change the name of the jar file to your jar file in the 3rd line. In my
FROM tells Docker to install the image from Docker
VOLUME specifies a directory outside the container, where Spring Boot creates working directories for Tomcat
ADD tells Docker to add the specified jar file to the image as app.jar
EXPOSE tells Docker to expose this port to the outside world.
ENTRYPOINT tells Docker to execute the app.jar file.
That is all you need.
Now go to the Terminal and cd to the project directory and execute the following commands.
1 2 3 |
docker build -t containerizedms . (this will build Docker image, change the last argument name as you like) docker run -d -p 8080:8080 containerizedms (this will create a container from above image and map port 8080 inside the container to 8080 of the host machine) docker ps (check if the container is running) |
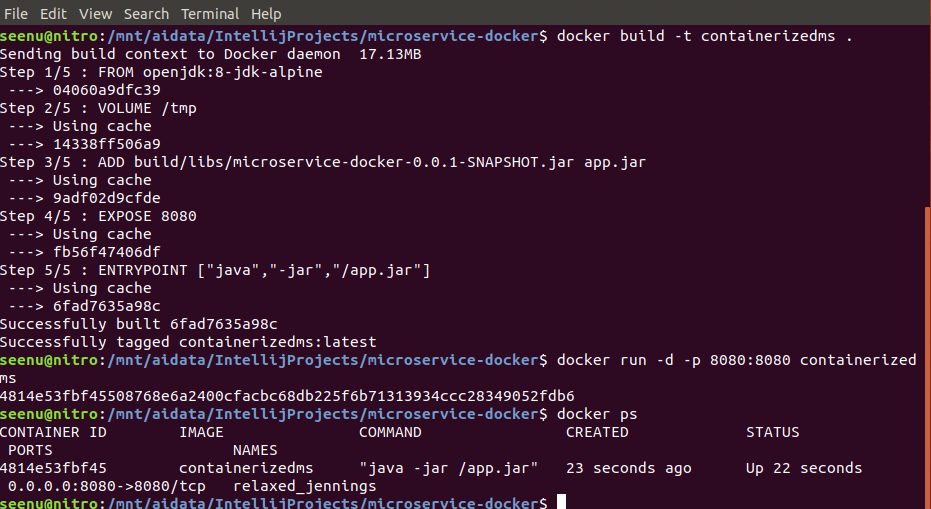
Click on http://localhost:8080 and you should see “My First Dockerized Microservice” in your
In the 2nd command -d tells Docker to run it as detached so your Terminal is not tied up. Port number on the left is host machine port, it can be any valid port. Docker container is an instance of an image. Think of an image as a Java class and a container as an instance of that class.
Once, you are done stop and remove containers with the following commands. Note that, each time you use Docker run, it creates a new container from the image.
1 2 |
docker ps (shows all the running containers along with container ids) docker rm <container id> (This will delete the container) |
Download Source code from Github.